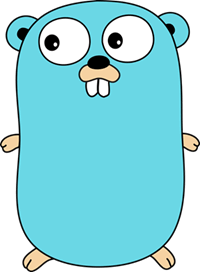
Master the basics and advanced features of the Go Programming Language.
If you want to learn Go, now is the perfect time! This tutorial is designed to help you get started with Go as quickly as possible. We’ll start with the basics and then dive into some of the more advanced features of the language.
Unlike other tutorials that only cover the basics like for-loops and if-statements, this tutorial will teach you how to harness the full power of Go’s concurrency model and interface-type systems.
Go is famous for being easy to learn but challenging to master. Through various programs, quizzes, and assignments, you’ll quickly grasp the language’s unique quirks.
Like any other programming language, the best way to learn Go is by writing code and building things. Therefore, this tutorial will give you many opportunities to do just that, encouraging you to work on your programs.
In this tutorial, you’ll:
- Understand the basic syntax and control structures of Go.
- Master the types in Go, especially if you are familiar with a dynamically typed language like JavaScript or Python.
- Organize your code effectively using packages.
- Apply Go’s concurrency model to build massively parallel systems.
- Use the Go runtime to develop and compile projects.
Master Go by starting the tutorial now!
Section 1. Getting Started with Go
- What is Go – Introduction to Go programming language.
- Install Go – Show you step-by-step how to install Go on your computer.
- Build a Hello World program in Go – Learn a lot of basic features of Go programming language via a simple Hello, World program.
Section 3. Control Flow
- If…else – Show you how to use the if…else statement to execute a code block based on one or more conditions.
- Switch – Learn how to execute a branch by comparing a value with multiple possible values.
- For loop – Execute a block of code repeatedly for a specified number of times or as long as a condition is true.
- For range loop – Iterate over the elements of a collection such as an array or a slice.
- Break – Stop a loop immediately
- Continue – Skip the remaining code of the current iteration and start a new one.
Section 4. Functions
- Functions – Define functions that encapsulate reusable logic and make the code more modular.
- Defining functions that return multiple values – Learn how to define functions that return multiple values.
- Functions are first-class citizens – Show you how to assign a function to a variable, pass a function to another function as an argument, and return a function from a function as a return value.
- Defer Functions – Guide how to defer a function execution after the surrounding function returns.
- Go Pointers and Pass by values – Help you understand Go Pointers and how functions use the “pass by value” mechanism.
Section 5. More Go Data Structures
- Arrays – Learn how to use arrays to hold a fixed number of elements with the same type.
- Slices – Explain slices which are views to arrays but provide dynamic size.
- Maps – Show you how to create a map that associates keys with values.
- Structs – Learn how to create a struct, which is a composite type that includes one or more fields.
Section 6. Methods & Interfaces
- Methods – Learn how to define methods on types including structs.
- Interface – Show you how to use interfaces to write more flexible code that accepts different types as long as they implement the same interface.
- Empty Interface: interface {}: Use empty interfaces to represent values of any type, which is quite flexible.
Section 7. Concurrency
- Goroutines – Learn how to use goroutines to run functions concurrently.
- Channels – Use channels to allow goroutines to exchange data.
- Select – Show you how to use the select statement to manage multi-channel operations and process the first available channel.
Section 8. Go Tools
- Set Up Live Reload for Go Programs with Air – Set up live reload for Go programs using the Air utility to boost your productivity and streamline the development workflow.
- Set Up Live Reload for Go Programs with Nodemon – Walk you through the steps to set up live reloading for a Go program using nodemon. This tutorial is optional if you’re uncomfortable working with Node.js and NPM.